Initialising the SDK
Before either of the FACEIT Game Client or Server SDK libraries may be used, they must first be initialised. The only functions that may be used before initialisation are logging functions, as useful log messages may be emitted if the initialisation process goes wrong. Other than this, no other feature functions will operate unless the SDK has been initialised.
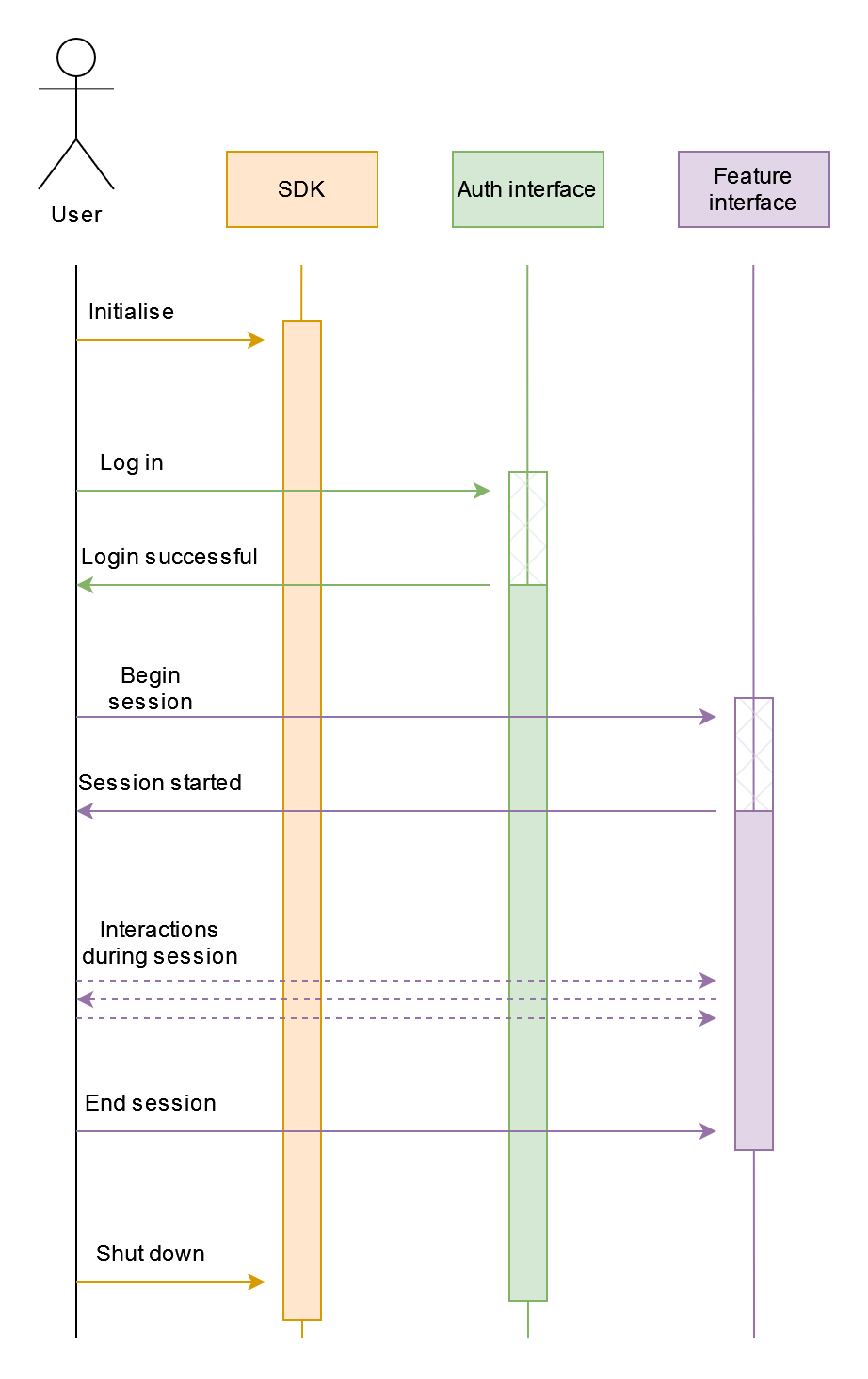
A typical SDK session will look something like the diagram above. The main steps are:
- The game initialises the FACEIT SDK, which sets up all interfaces for use.
- The current user logs in, and is authenticated with FACEIT. Before this step, no interfaces other than Auth or Logging will function.
- The game begins a session with the desired feature interface.
- The game continues to interact with this interface throughout the session.
- Once the game no longer needs to use the feature, it ends the session.
- When the game application terminates, it shuts down the FACEIT SDK.
The code in the sections below refers to the FACEIT Game Client SDK. For the server SDK, simply replace the appropriate headers, and function prefixes or namespaces, with those referring to the server.
Initialisation
info
The FACEIT Unreal plugins are set up as game instance subsystems, so do not need to be initialised manually. Once your game instance has started, the FACEIT Unreal plugins will be ready for use.
To initialise the SDK, call the Initialise()
function. This function returns a boolean indicating whether initialisation was successful.
- C++
- C#
#include "FACEITGameClientSDK/LibInit.h"
bool initialisedSuccessfully = FGCSDK_Initialise();
using FACEITGameClientSDK;
bool initialisedSuccessfully = Library.Initialise();
In the unlikely event that initialisation fails, call GetInitialisationError()
to obtain a string description of what went wrong.
- C++
- C#
#include "FACEITGameClientSDK/Utilities/StringHelpers.h"
std::string errorString = FGCSDK_ToString(&FGCSDK_GetInitialisationError);
string errorString = Library.GetInitialisationError();
Once the SDK is no longer required for use, it should be shut down. This will terminate any sessions that are currently active, and will clean up all resources that are currently in use.
- C++
- C#
FGCSDK_ShutDown();
Library.ShutDown();
Note that although active sessions will be terminated when shutting down the SDK, FACEIT recommends that sessions are ended individually by calling the relevant EndSession()
functions. This ensures that these active sessions end gracefully, and are able to close down any external connections correctly.
info
Shutting down the SDK does not clear the logging callback. To do this, call ClearLogMessageCallback()
in the Logging interface.
Polling
Throughout its lifetime, the FACEIT SDK must be polled regularly in order to continue to operate.
- C++
- UE4
- C#
#include "FACEITGameClientSDK/LibInit.h"
FGCSDK_Poll();
#include "FACEITUnrealClientPlugin/UFUCP_Lib.h"
UFUCP_Lib::Poll();
using FACEITGameClientSDK;
Library.Poll();
Control of the polling frequency is managed entirely by the game developer, but FACEIT recommends that the SDK be polled at least ten times per second. Poll()
is expected to be called once per game frame under most circumstances.
Certain SDK interfaces operate using callback functions provided by the user. These callbacks are called in response to certain events, eg. receiving a response to a web request. Callbacks are only ever executed as a result of a call to Poll()
, and Poll()
should never be called from within a currently executing callback.
When Poll()
is called, any callbacks that are executed will be run on the same thread that called Poll()
.