The FACEIT SDK
The FACEIT SDK can be downloaded by game publishers and game developers by creating an account and logging into the FACEIT Labs portal.
The FACEIT SDK provides an open and modular set of online services for game development. The SDK aims to be easy to use, scalable, and maintainable. It is designed to be accommodating of any game engine, any store, or any major platform.
Leveraging our consumer knowledge with the FACEIT Platform and Media Events at ESL, FACEIT Labs brings this experience into the tools we offer and are planning to offer to our game developers.
If you are experiencing issues accessing the portal, please let your FACEIT Support contact know.
SDK Fundamentalsβ
The business logic of the FACEIT SDK is written in C++. This provides a stable, cross-platform foundation for game developers to use, regardless of their choice of game engine or implementation.
In addition, the SDK includes integrations with specific game engines (eg. Unreal or Unity) via plugins, for developer convenience. These releases are based on the same core implementation as the generic C++ release.
Versioningβ
The FACEIT SDK guarantees compatibility with games using previous SDK versions, by following a semantic versioning convention. An increase in the major or minor version number implies that the external interface to the SDK has changed; an increase in the patch version number guarantees no change in the external interface.
Using the FACEIT SDK in a Game Projectβ
The FACEIT SDK is divided into two libraries, which respectively provide functionality for use with game clients and game servers.
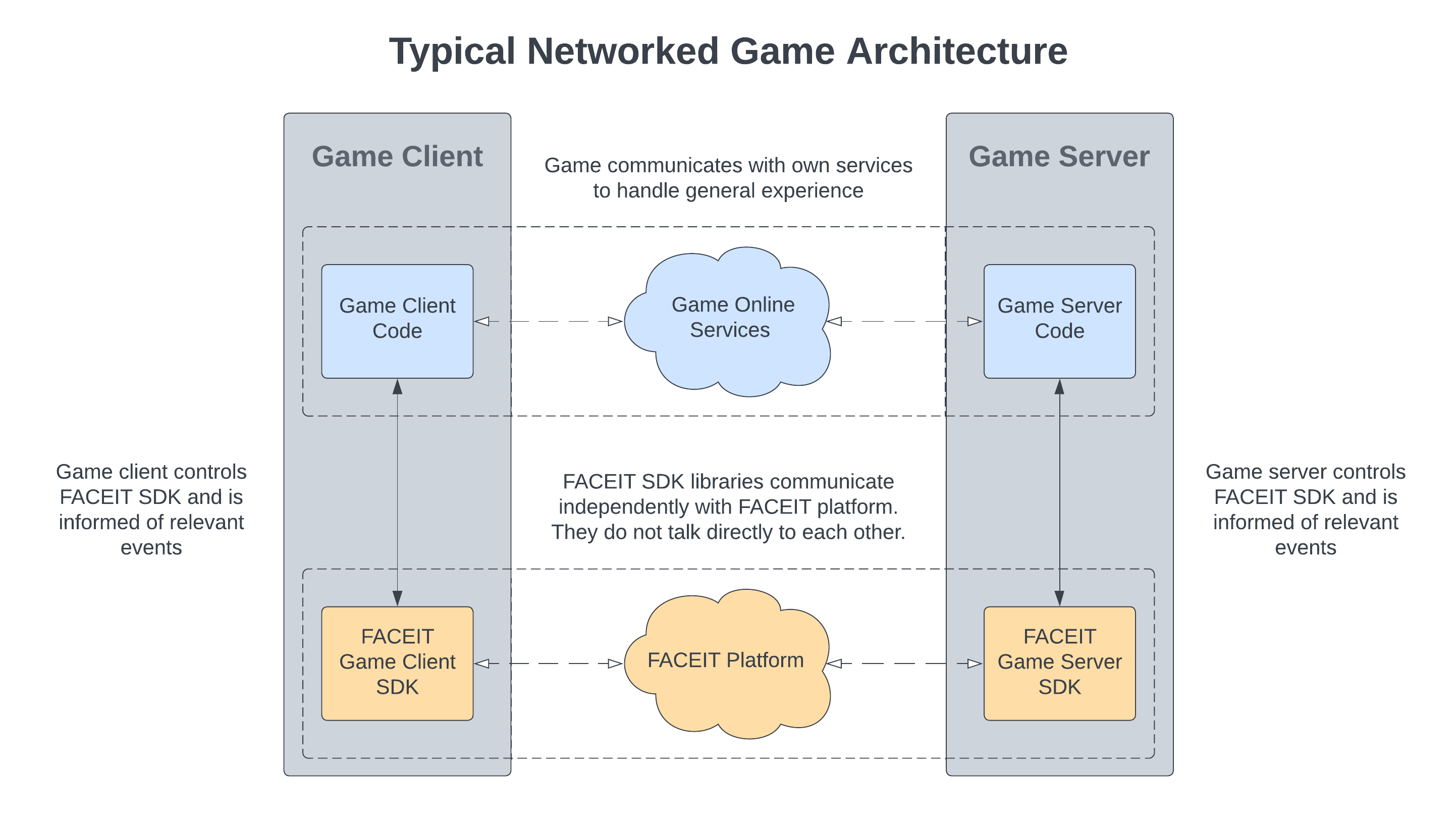
The FACEIT SDK libraries both communicate with the FACEIT backend for their feature functionality. The game client and game server applications control the SDK libraries, and may respond to events by registering appropriate callbacks.
To use the FACEIT SDK libraries with a game project, add them based on the following configuration.
Pure C++β
Application Type | Link Against | Library Paths | Include Paths |
---|---|---|---|
Windows Client | FACEITGameClientSDK.dll FACEITGameClientSDK.lib | FACEITGameClientSDK/bin FACEITGameClientSDK/lib | FACEITGameClientSDK/include |
Windows Server | FACEITGameServerSDK.dll FACEITGameServerSDK.lib | FACEITGameServerSDK/bin FACEITGameServerSDK/lib | FACEITGameServerSDK/include |
Linux Client | libFACEITGameClientSDK.so | FACEITGameClientSDK/lib | FACEITGameClientSDK/include |
Linux Server | libFACEITGameServerSDK.so | FACEITGameServerSDK/lib | FACEITGameServerSDK/include |
Unreal Projectβ
Adding the specified plugin to your project should automatically make the relevant headers and libraries available.
Application Type | Plugin | Supported Platforms |
---|---|---|
Game Client | FACEITUnrealClientPlugin | Windows, Linux (*) |
Game Server | FACEITUnrealServerPlugin | Windows, Linux |
(*) Certain features such as FACEIT Anti-Cheat are not supported on Linux.
Unity Projectβ
The FACEIT SDK C# libraries are compatible with the Unity game engine. They support building with either IL2CPP or Mono.
caution
Unity Engine has a known problem using C# .dll libraries in combination with IL2CPP with some engine versions. To mitigate this, compile the SDK C# libraries from source in your project.
Application Type | Pre-Compiled | Source |
---|---|---|
Windows Client | FACEITClientCSharp.dll | FACEITGameSDK_CSharp/FACEITClientCSharp/Public FACEITGameSDK_CSharp/FACEITClientCSharp/Private |
Windows Server | FACEITServerCSharp.dll | FACEITGameSDK_CSharp/FACEITServerCSharp/Public FACEITGameSDK_CSharp/FACEITServerCSharp/Private |
Naming Conventions and Compatibilityβ
All FACEIT SDK headers and interfaces are appropriately named according to their library (client or server), and the service they refer to.
Pure C++β
The C++ library headers are written to be C-compliant, for portability. As formal namespaces are not supported by the C language, functions are named following the convention below:
[Libray]_[Interface]_[Operation](...);
Functions prefixed with FGCSDK_
belong to the FACEIT Game Client SDK, and functions prefixed with FGSSDK_
belong to the FACEIT Game Server SDK.
All types used within public interface functions are explicitly defined to refer to portable underlying C types, or are custom structs built from these portable types. The compiler is expected to support stdbool.h
and stdint.h
.
Unrealβ
Formal C++ namespaces do not work well with the Unreal Header Tool, so Unreal classes and functions are also named using specific naming conventions. Client plugin names begin *FUCP
, referring to the FACEIT Unreal Client Plugin, and server plugin names begin *FUSP
, referring to the FACIET Unreal Server Plugin. The first character of the name reflects the type being used, as per Unreal conventions.
Unityβ
Client SDK types and functions are contained within the FACEITGameClientSDK
namespace, and server SDK types and functions are contained within the FACEITGameServerSDK
namespace.
Error Handlingβ
Many FACEIT SDK functions return a result code. This code indicates whether an operation has completed successfully or not. Result codes should always be checked in order to verify the success of a function call.
Certain asynchronous SDK functions will return a result code from the function call, and additionally will supply a result code on the functionβs callback once the asynchronous operation has completed. The result code returned from the function represents whether the operation was able to be initiated, and the result code in the callback represents the success of the overall call. If the result code returned from the function is OK, the asynchronous callback is guaranteed to be called later; if the result code represents an error, the asynchronous callback will not be called.
Stringsβ
The FACEIT SDK passes strings across library boundaries as C-style pointers, to avoid compiler ABI issues when using C++ types. All strings in the FACEIT SDK are encoded as UTF-8.
Any functions which return strings require the user to pass a buffer to copy the string into. By convention, these functions will return the length of the string regardless of what buffer is passed in, so may be called with a null buffer as an argument in order to determine the length of the string by itself.
Although the entirety of the SDK interfaces are C-compatible, C++ string helper functions are present in the Utilities/StringHelpers.h
header. These functions allow SDK string buffers to be easily converted to a std::string
:
// Before:
#include "FACEITGameClientSDK/Log.h"
// A three-step process: determine the buffer size, allocate the buffer,
// and write the string to the buffer.
// For this example, the buffer is assumed to be deleted elsewhere.
FGCSDK_Size bufferSize = FGCSDK_GetLogLevelString(nullptr, 0, FGCSDK_LOG_INFO) + 1;
FGCSDK_Char* buffer = new FGCSDK_Char[bufferSize];
FGCSDK_GetLogLevelString(buffer, bufferSize, FGCSDK_LOG_INFO);
// After:
#include "FACEITGameClientSDK/Log.h"
#include "FACEITGameClientSDK/Utilities/StringHelpers.h"
// Simply pass the address of the function, plus any required arguments.
std::string logLevelString = FGCSDK_ToString(&FGCSDK_GetLogLevelString, FGCSDK_LOG_INFO);
Memory Allocationβ
Most of the types exposed by the FACEIT SDKβs interfaces are primitives and are passed to the user by value. However, for more complex types like strings or structs, any of these types exposed by pointer or reference are considered to have their lifetime owned by the FACEIT SDK. If you wish to use these data items at a later time, you must make a copy of them locally.
Thread Safetyβ
The FACEIT Game Client SDK and Game Server SDK are not currently built to be accessed by multiple threads. If multiple threads may call into the SDK, it is the responsibility of the game developer to ensure that no two threads call functions simultaneously. It is acceptable to interact with the SDK on a dedicated worker thread, provided the above restrictions are met.