How to Get Started
info
As a prerequisite, it is required your game fully supports the Server Match Life Cycle integration to use the Matchmaking integration. Reach out to your technical integration contact if you have any questions.
The Client Matchmaking interface exposes the FACEIT matchmaking service to game developers. Using the interface, a player can join and leave any matchmaking queues set up for the game, and will be notified when matched with other players.
Availability
Domain | Interface Presence |
---|---|
C++ | Client SDK v6.0.0+ |
Unreal | Client SDK v6.0.0+ |
C# | Client SDK v4.0.0+ |
Overview
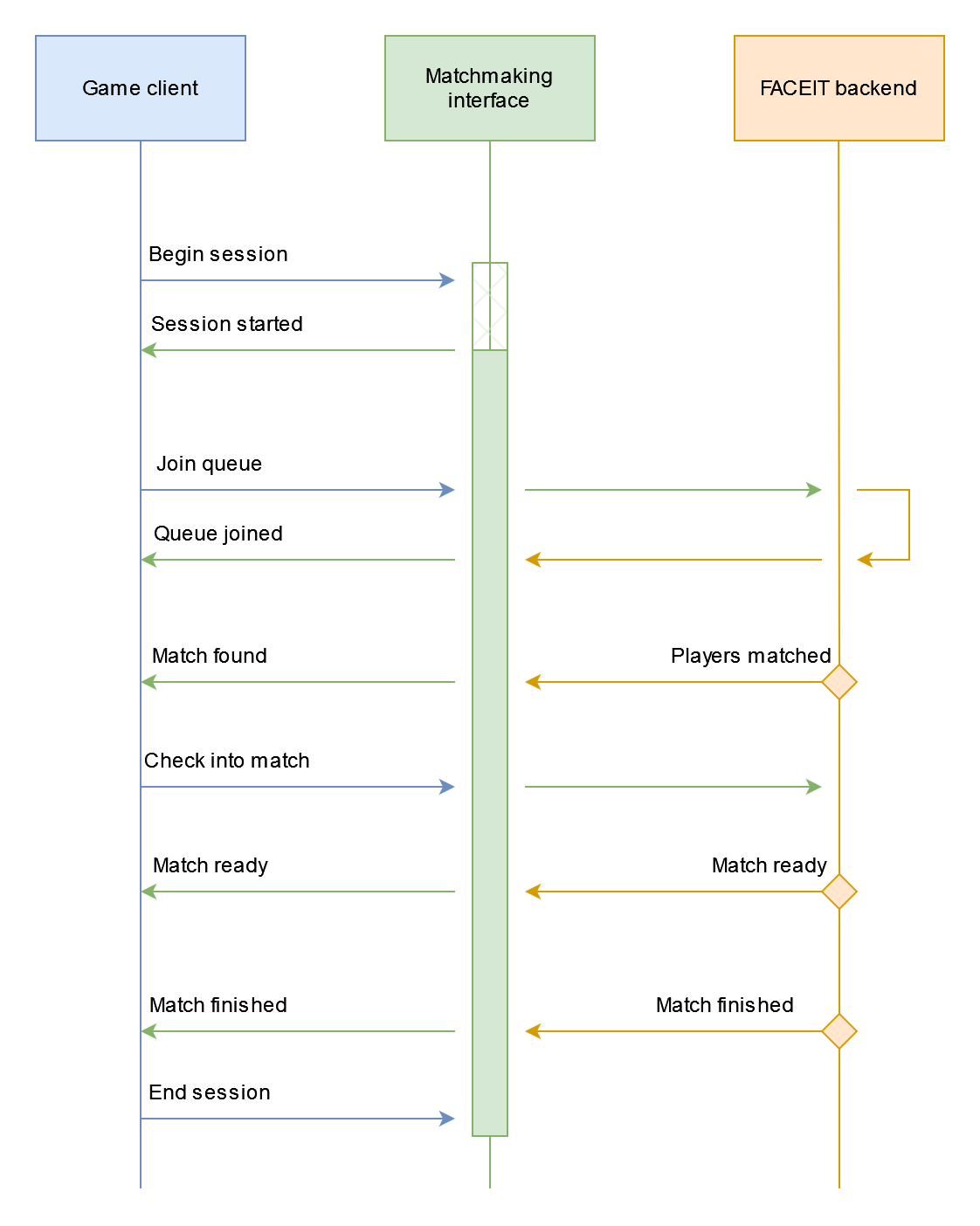
The Matchmaking interface sits between a game client and the FACEIT backend. It presents information to the game, such as which matchmaking queues are available for players to join, and notifies the game of queue- and match-related events that occur on the FACEIT platform.
Follow these steps to use the Client Matchmaking interface in your game:
- Start the FACEIT Client SDK and authenticate the user.
- Begin a session with the Client Matchmaking interface. The session holds a particular configuration for matchmaking, including callbacks for events, and settings like match auto check-in.
- Use the interface to get a list of available queues, and join one or more of these queues.
- When a match is found for the current user, the
OnMatchFound
event is fired. If auto check-in is not enabled, use the interface to check the user into the current match. - When the match is ready to play, connect information is provided on the
OnMatchReady
event. Connect the user's game client to the server using the provided information. - When the match finishes, the
OnMatchFinished
event is fired. - When matchmaking functionality is no longer required, use the interface to end the matchmaking session.
Event Summary
The Client Matchmaking interface exposes the following events. These events may be handled by providing callbacks to the interface when beginning a session.
OnUserJoinedQueue
Called when a user joins a queue. This may be in response to a JoinQueue()
call to the Matchmaking interface, or it may be triggered by some other cause (eg. the player's team leader added their team to a queue).
Parameters:
- ID of the queue that was joined.
OnUserLeftQueue
Called when a user leaves a queue. This may be in response to a LeaveQueue()
call to the Matchmaking module, or it may be triggered by some other cause (eg. the player was automatically removed from a queue because they matched with other players).
Parameters:
- ID of the queue that was left.
OnUserStatusChanged
Called when the current user's matchmaking status changes. This status represents the user's availability for matchmaking.
Parameters:
- New status of the user. This may be one of the following:
- Available: The user is available to join queues or matches.
- In Queue: The user is part of at least one queue for the current game.
- In Match: The user is playing in a match for the current game.
- Unavailable: The user is playing in a match for a different game, and so cannot currently participate in matchmaking for this game.
OnMatchFound
Called when a match for the current user is found. The match must be checked into by all players in order for it to proceed.
Parameters:
- Whether auto check-in is enabled. If true, match check-in will be performed automatically; if false,
CheckIntoCurrentMatch()
must be called to perform check-in.
OnMatchReady
Called when a match the user is a part of is ready for play.
Parameters:
- Connection information. This information is provided as a JSON string, and is structured as per the game's configuration in the FACEIT Game Studio.
OnMatchFinished
Called when a match the user is a part of has finished.
Parameters:
- State that the match finished in. This may be one of:
- "FINISHED": The match finished normally.
- "ABORTED": The match was aborted because not all players joined.
- "CANCELLED": The match was cancelled by an administrator.
- "NO_CHECKIN": The match never became ready because not all players checked in.
OnQueueUpdated
Called when the status of a queue is updated.
Parameters:
- ID of the queue that was updated.
- Whether the queue is now open or closed.
Method Summary
The Client Matchmaking interface exposes the following methods.
BeginSession
Begins a matchmaking session, providing the configuration for the session.
Parameters:
- Session settings. These include:
OnUserJoinedQueue
callbackOnUserLeftQueue
callbackOnUserStatusChanged
callbackOnMatchFound
callbackOnMatchReady
callbackOnMatchFinished
callbackOnQueueUpdated
callback- Whether auto check-in should occur
EndSession
Ends an existing matchmaking session.
IsSessionActive
Returns whether there is currently an active matchmaking session ongoing.
GetUserStatus
Returns the current user status (Available, In Queue, In Match, or Unavailable).
GetQueues
Returns a list of queues for the current game. For each queue, the following attributes are returned:
- Queue ID
- Queue name
- Size of teams within matches that this queue produces
- Maximum allowed size for any party that joins this queue
- Game mode that this queue caters for
- Whether this queue contains the current user
GetQueuesContainingUser
Returns a list of queues for the current game that contain the current user. The data format is the same as that returned by GetQueues()
.
JoinQueue
Adds the current user to a queue, specified by ID. The queue must be for the current game. Solo and party queues are supported.
LeaveQueue
Removes the current user from a queue, specified by ID. The queue must be for the current game. Solo and party queues are supported.
HasCurrentMatch
Returns whether a match currently exists for the user. A match is considered "current" if:
- The match is for the current game
- The match has been "found" (ie.
OnMatchFound
has been fired) - The match has not finished (ie.
OnMatchFinished
has not been fired)
If a current match exists, functions that operate on the current match may be invoked. If no current match exists, these functions will fail if invoked.
CheckIntoCurrentMatch
Checks the user into the current match. If the session is configured to perform check-in automatically, calling this function is not required. Otherwise, this function must be called for the current user before the match check-in time expires, or the match will end in a "NO_CHECKIN" state.
GetCurrentMatchConnectInformation
Returns the connect information for the current match, if the match is in a state where players may connect. The connect information is identical to that which would have been provided on the OnMatchReady
event.
GetCurrentMatchConnectInformation()
may be called to obtain the connect information in cases where an OnMatchReady
event may have been missed, eg. if a match was already ready before BeginSession()
was called. If no match is ready, an empty string is returned.